You can store functions in one or more files outside your main script. In order to utilize these
functions, you must have an “include” statement, then you can just call them as you would any other
locally defined function. The include line does not actually have a syntax, it simply reads in additional
functions.
Here we are adding the file install_lib, which contains a series of functions.
. src/install_libs (note the leading ‘dot space’ meaning ‘look in this file plus…’).
INTERIGATING FOR VARIABLES
Although the BASH shell is included in most Unix and Linux systems, some of the system commands
or utilities are located in different directories. If you want your script to be truly portable, you must
take this into account. Below is one of many ways to do it.
We read a file if the command names, formatted one command per line.
It’s contents may look like this:
rm
grep
sed
awk
Then we search for them and save the output to an include file:
(the shortfall here is the ‘which’ command must be available, a more complex method is used in the
Flac Jacket scripts)
while read CMD_VAR
do
CMD_NAME=”`echo $CMD_VAR|` which tr` [:lower:] [:upper:]`”
echo “${CMD_NAME}=`which ${CMD_VAR}`” >> command_include_file
done < command_list.txt
A simple script to send a monthly email reminder
#!/bin/bash
#
# send email reminder to visit yi monthly
# run from cron monthly
/usr/bin/printf “Time to update yi.orgnnthe url is http://www.whyi.org/admin/n”|/bin/mail s “Update
YI” pete
This script backs up a local unix/linux system to a Windows server
#!/bin/bash
#
# script to backup local files on remote system via ssh pubkey athentication.
# both systems must be setup for sshkey auth.
# June 26, 2000. Pete Nesbitt
# file to read list of source dirs from.
SOURCES=/etc/backup_src.txt
EXCLUDE=/tmp/tar_exclude.tmp
# target host and dir
TARGET_HOST=192.219.60.22
TARGET_DIR=$TARGET_HOST:e:/bupnesbitt
while read LINE
do
TARFILE=”/tmp/`echo $LINE| sed e s/’/’/_/g `.tar”
find `echo $LINE` type s > $EXCLUDE
find `echo $LINE` type p >> $EXCLUDE
# check which tar, the P will either GNU=”not remove the leading /” or Solaris=”don’t add a trailing
/”
/bin/tar cPf $TARFILE X $EXCLUDE $LINE
/bin/gzip 9 $TARFILE
# next line forces “open ssh” as that is what the server uses, open & com don’t play well.
su backup c “/usr/bin/scp $TARFILE.gz $TARGET_DIR/”
rm f $TARFILE.gz
done < $SOURCES
example of a FUNCTION
error_1 ()
{
echo ” ERROR: There is a “${ERR_TARGET}” directory, but you can not write to it.”
error_mesg_perm
echo “”
exit 1
}
######
error_2 ()
{
echo ” ERROR: There is a file named “${ERR_TARGET}”, but it does not appear to be a
directory.”
echo ” Remove or rename the “${ERR_TARGET}” file, or choose a different location, and try
again.”
echo “”
exit 1
}
they can be called like this:
(note the locally defined “ERR_TARGET” as well as the function call such as “error_1”)
# does the thumbnails exist, if so is it a dir, if so can we write to it
ERR_TARGET=”thumbnails”
if [ e $THUMBNAILS ]; then
if [ d $THUMBNAILS ]; then
# make sure we can write to it
if ! [ w $THUMBNAILS ]; then
error_1
fi
else
error_2
fi
else
mkdir $THUMBNAILS
#run a test for success
if [ d $THUMBNAILS ]; then
if ! [ w $THUMBNAILS ]; then
error_3
fi
fi
fi
EOF
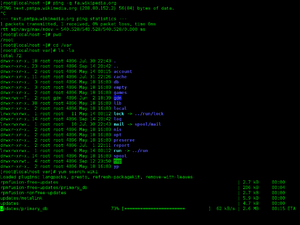
Leave a Reply